In a previous post I showed that I am using a KML file as input into GMaps.js to put a bunch of points on a map for my travels site. This worked great, but I really want to do some marker clustering since too many points is pretty bad looking as can be seen below.
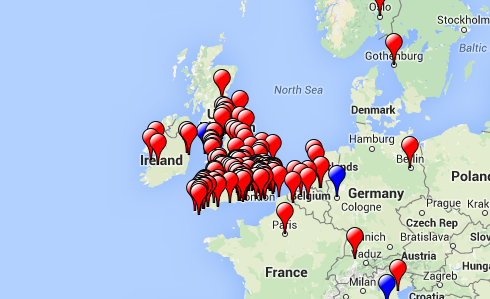
I’d much rather do some clustering and expand out to multiple points when you zoom in like here:
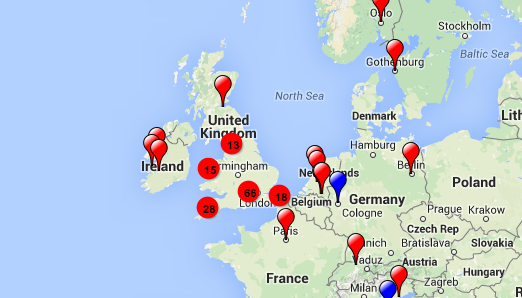
Turns out there are a few libraries for this already, I started out with one called Marker Clusterer but ended up with a improved version of this called Marker Clusterer Plus. And GMaps.js supports cluster libraries natively so should be easy, right?
Turns out the way Google maps loads KML files is done using a layer over the map and the points just are not accessible to any libraries, so the cluster libraries does nothing. Ok, so back to drawing points using my code.
I added a endpoint to the app that emits my points as JSON:
[ {"type":"visit", "country":"Finland", "title":"Helsinki", "lat":60.1333, "popup_html":"<p>\n<font size=\"+2\">Helsinki</font>\n<hr>\nBusiness trip in 2005<br /><br />\n\n</p>\n", "comment":"Business trip in 2005", "lon":25, "icon":"/markers/marker-RED-REGULAR.png"} ] |
Now adding all the points and getting them clustered is pretty easy:
<script type="text/javascript"> var map; function addPoints(data) { var markers_data = []; if (data.length > 0) { for (var i = 0; i < data.length; i++) { markers_data.push({ lat: data[i].lat, lng: data[i].lon, title: data[i].title, icon: data[i].icon, infoWindow: { content: data[i].popup_html }, }); } } map.addMarkers(markers_data); } $(document).ready(function(){ infoWindow = new google.maps.InfoWindow({}); map = new GMaps({ div: '#main_map', zoom: 15, lat: 0, lng: 20, markerClusterer: function(map) { options = { gridSize: 40 } return new MarkerClusterer(map, [], options); } }); points = $.getJSON("/points/json"); points.done(addPoints); }); </script> |
This is pretty simple, the GMaps() object takes a markerClusterer option that expects an instance of the clusterer. I fetch the JSON data and each row gets added as a point. Then it all just happens automagically. Marker Clusterer Plus can take a ton of options that lets you specify custom icons, grid sizes, tweak when to kick in clustering etc. Here I am just setting the gridSize to show how to do that. In this example I have custom icons used for the clustering, might blog about that later when I figured out how to get them to behave perfectly.
You can see this in action on my travel site. As an aside I’ve taken a bit of time to document how the Sinatra app works and put together a demo deployable to Heroku that should give people hints on how to get going if anyone wants to make a map of their own.